How to develop Scalable Programs as a Developer By Gustavo Woltmann
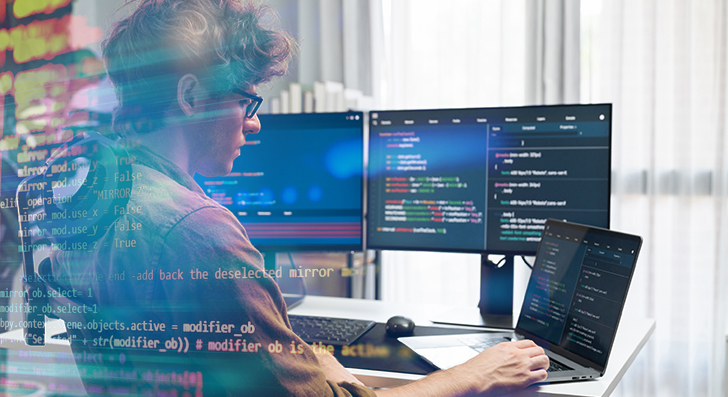
Scalability usually means your application can handle advancement—far more consumers, more details, plus much more website traffic—with no breaking. As being a developer, setting up with scalability in your mind saves time and stress later on. Right here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later—it ought to be aspect of one's system from the beginning. Quite a few programs are unsuccessful once they improve quick simply because the first style can’t cope with the extra load. Like a developer, you might want to Feel early regarding how your method will behave stressed.
Begin by coming up with your architecture to become versatile. Stay clear of monolithic codebases the place everything is tightly linked. As a substitute, use modular design or microservices. These styles break your app into more compact, unbiased parts. Every single module or company can scale on its own with no influencing The full procedure.
Also, consider your database from day just one. Will it need to deal with 1,000,000 people or simply just a hundred? Select the suitable style—relational or NoSQL—based on how your information will expand. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them nonetheless.
One more critical place is to stay away from hardcoding assumptions. Don’t generate code that only functions underneath latest disorders. Give thought to what would happen When your consumer base doubled tomorrow. Would your app crash? Would the database decelerate?
Use design styles that aid scaling, like information queues or celebration-driven units. These assistance your application cope with additional requests devoid of receiving overloaded.
If you Construct with scalability in mind, you're not just preparing for fulfillment—you might be cutting down foreseeable future head aches. A nicely-planned procedure is simpler to maintain, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the right databases can be a important part of developing scalable purposes. Not all databases are created the identical, and utilizing the Erroneous one can gradual you down as well as trigger failures as your application grows.
Get started by comprehension your information. Can it be hugely structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically solid with relationships, transactions, and regularity. They also guidance scaling methods like browse replicas, indexing, and partitioning to deal with extra targeted traffic and data.
When your info is a lot more flexible—like consumer activity logs, product or service catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured information and might scale horizontally extra very easily.
Also, take into consideration your study and produce styles. Have you been executing lots of reads with less writes? Use caching and browse replicas. Are you currently dealing with a major create load? Investigate databases which can handle large produce throughput, or even occasion-based mostly data storage methods like Apache Kafka (for short term facts streams).
It’s also good to think ahead. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them signifies you received’t will need to modify later.
Use indexing to speed up queries. Steer clear of pointless joins. Normalize or denormalize your info dependant upon your entry designs. And constantly monitor database overall performance as you develop.
In brief, the proper database depends upon your app’s structure, velocity requires, and how you expect it to grow. Take time to select sensibly—it’ll help save many difficulties later on.
Optimize Code and Queries
Quick code is essential to scalability. As your app grows, every compact hold off adds up. Badly written code or unoptimized queries can decelerate effectiveness and overload your process. That’s why it’s crucial that you Construct effective logic from the start.
Start by crafting clear, straightforward code. Stay away from repeating logic and remove just about anything unneeded. Don’t choose the most complex Option if an easy 1 functions. Keep the features short, concentrated, and simple to check. Use profiling equipment to locate bottlenecks—sites the place your code requires much too prolonged to run or uses an excessive amount memory.
Subsequent, evaluate your database queries. These normally sluggish matters down a lot more than the code itself. Be sure each question only asks for the data you truly require. Prevent Choose *, which fetches anything, and rather pick out particular fields. Use indexes to hurry up lookups. And stay clear of carrying out a lot of joins, Particularly across big tables.
When you notice the identical details getting asked for many times, use caching. Shop the final results quickly using equipment like Redis or Memcached therefore you don’t really need to repeat highly-priced functions.
Also, batch your database operations if you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and tends to make your app far more successful.
Make sure to test with massive datasets. Code and queries that get the job done great with 100 records may crash whenever they have to manage one million.
Briefly, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when needed. These measures support your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to deal with far more end users plus much more website traffic. If anything goes through one server, it will quickly turn into a bottleneck. That’s where load balancing and caching come in. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout multiple servers. Instead of a person server executing the many operate, the load balancer routes consumers to various servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for exactly the same information and facts yet again—like a product page or maybe a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There's two frequent types of caching:
one. Server-side caching (like Redis or Memcached) merchants data in memory for rapid access.
two. Client-facet caching (like browser caching or CDN caching) retailers static data files near to the person.
Caching decreases databases load, improves velocity, and tends to make your application more successful.
Use caching for things that don’t modify normally. And often make certain your cache is up-to-date when data does adjust.
To put it briefly, load balancing and caching are straightforward but highly effective tools. Collectively, they assist your app manage additional customers, remain rapid, and recover from difficulties. If you plan to expand, you require both of those.
Use Cloud and Container Resources
To create scalable purposes, you need resources that allow your application mature easily. That’s in which cloud platforms and containers can be found in. They offer you adaptability, decrease setup time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and expert services as you would like them. You don’t have to buy hardware or guess long term capability. When site visitors will increase, it is possible to insert get more info additional methods with just a couple clicks or quickly applying vehicle-scaling. When traffic drops, you can scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and security applications. You could deal with making your application as an alternative to controlling infrastructure.
Containers are Yet another important Device. A container packages your application and all the things it ought to run—code, libraries, settings—into one device. This causes it to be simple to maneuver your application among environments, from your notebook to your cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app utilizes multiple containers, instruments like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your app crashes, it restarts it automatically.
Containers also enable it to be simple to separate portions of your app into expert services. You'll be able to update or scale parts independently, and that is great for overall performance and trustworthiness.
In brief, applying cloud and container resources usually means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you want your app to mature without having restrictions, begin working with these tools early. They preserve time, decrease chance, and help you remain centered on developing, not repairing.
Observe Every thing
In case you don’t monitor your application, you gained’t know when points go wrong. Monitoring aids the thing is how your application is performing, spot troubles early, and make improved decisions as your application grows. It’s a vital part of creating scalable programs.
Get started by monitoring basic metrics like CPU usage, memory, disk Room, and response time. These inform you how your servers and expert services are doing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—watch your application much too. Regulate how much time it takes for consumers to load web pages, how often problems come about, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or a service goes down, you should get notified immediately. This allows you take care of difficulties speedy, normally in advance of end users even recognize.
Monitoring is also practical any time you make alterations. Should you deploy a brand new feature and find out a spike in problems or slowdowns, you are able to roll it again ahead of it triggers real destruction.
As your app grows, visitors and details enhance. Without having checking, you’ll overlook signs of trouble until eventually it’s also late. But with the right instruments in position, you continue to be on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your procedure and ensuring it really works effectively, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest applications require a robust foundation. By coming up with cautiously, optimizing correctly, and using the proper applications, you'll be able to Establish apps that increase effortlessly with out breaking stressed. Get started tiny, Assume big, and Construct good.